Skip to main content
Live from PDC 2008: A First Look at Windows 7
Introduction to Threat Modeling
Part of my work at Microsoft is ensuring that we get a high quality threat model tool out to the public. I want to take this post to introduce developers and architects to the idea. Using the beta version of the SDL Threat Modeling Tool v3 located at https://msdn.microsoft.com/en-us/security/dd206731.aspx, I created the following diagram. You can do this with any drawing tool, but using the SDL tool is much easier and much faster. Note that what I am going to discuss might deviate from the official tutorial and introduction articles since this is my own perspective on threat modeling.
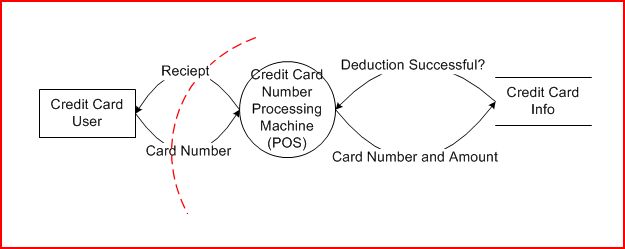
Here, a credit card user gives his card to a POS(point of sales) device, which then gives the card number and amount to be deducted to some credit card database system. The system either deducts that amount and tells POS deduction succeeded or tells POS the deduction failed because the limit has been reached. Finally, POS produces a receipt. In between the user and the POS, we have the dotted red line, which is a trust boundary, marking the fact that anything could come from the user side, and our system to the right should take that data with a grain of salt.
What I am describing is not a software system, traditional targets of threat modeling, but a common physical and software system that exists in the real world. It will demonstrate the idea of threat modeling nevertheless. Given the system above, what are all possible attacks? If we have a list, then we can go step by step and check each of the items off the list to prevent all possible attack against the system design. Note that this is not a claim against 0 vulnerability system. Vulnerabilities can still exist in the implementation.
An example. The card number going from the user to the POS can have following threats against it.
- Tampering. Did the cashier at any point change the number? How do we verify that the card number handed by the user is the one entered into the system? Do we require user confirmation? This is probably not a big threat in the real world since people wouldn't mind if cashier used the wrong number.
- Information Disclosure. Can other people read that numbers off the card? Information disclosure is when the information can be read by an unauthorized party.
- Denial of Service. Is there a way for the person to quick swipe his card at a POS 100 times in a row and cause the POS to crash?
If you entered the above system into the SDL Threat Modeling Tool v3, these threat types would automatically be generated by the tool. All of a sudden, we have covered 3 different types of attacks possible against our card number and POS. There are of course more, but for the purpose of this post, I've only looked at that small portion of our system. Using this methodology, we can solve a lot of security risk at design time!
Investment
I bought some citi stock at around $2.7. Now it's all the way up to $4 in around a week. Woot! Now the question is whether Fannie Mae and Freddie Mac would also be good investments. They were both valued at $60 at one time. Now they're both down to dollar values. Yet their functionality/monopoly remains. Just because people overinvested in the housing market, does that mean in a cooler market, fannie/freddie are only worth 1/60th of their older value? Anyway, this is certainly not my forte, but intuition tells me they might be good picks. Let's see what happens a year or two down the line.
Leaving Microsoft
This will be my last technet/msdn blog. All my future blog posts will be at https://softienerd.blogspot.com/.
Max Int Min Int - a Common Coding Mistake and a Sample Microsoft Interview Question
Write the function atoi, a string to integer converter.
Write the function itoa, an integer to string converter.
These are problems given to a lot of entry level interview candidates at Microsoft. I want to talk about just one aspect of this type of problem that most people do not get(maybe even the interviewer)! This subtle yet valid trap actually can happen a lot in real life coding situations.
Depending on how you designed your answer, you might eventually come down to a path where the number you want to eventually return is a negative number, but you are keeping it as a positive integer until the last second, when you either multiply it by -1 or add the positive number to the end of a string with '-' char. There is a subtle trap here. What if the number passed in is the maximum negative integer, or rephrased, the minimum int possible. I'll end the suspense right now, the absolute value of max int is smaller than the absolute value of min int. Ie Take a signed byte. The max signed byte value would be 2^7-1, but the min int value would be -2^7. If you think about it, with 8 binary digits, you can fit 2^8 distinct values in. So if the positive side contains 2^7-1 numbers, and one more for the 0, the negative side must contain 2^7 numbers. To illustrate:
00000000 - 0
01111111 - max signed byte, 2^7-1
11111111 - 2's complement -> 00000001
10000000 - 2's complement -> 10000000
In summary, max int != - min int
Update: Read an excellent XKCD episode that contained the same concept at https://xkcd.com/571/.
NoScript
On my laptop, I use firefox and an extension called NoScript. What NoScript does is blocking general javascripts from running. Apparently, it whitelist itself so that Ads that require javascript on its own website can run. Not only that, it went further by tampering with another Firefox extention called Adblock Plus so that Adblock Plus would not block NoScript's Ads. Here is the apology letter sent out to the Mozilla Community by Noscript author.
https://hackademix.net/2009/05/04/dear-adblock-plus-and-noscript-users-dear-mozilla-community/
Anyway, I do recommend this extension if you have firefox. But go ahead and modify the default whitelist once you install it.
On Windbg and Symbols
Every once a while, a developer(SDE) or a tester(SDET) will find himself needing to look at dump files or debug a process. Here's what each debugger technology out there will support:
Feature | KD | NTSD | WinDbg | Visual Studio .NET |
| | | | |
Kernel-mode debugging | Y | N | Y | N |
User-mode debugging | | Y | Y | Y |
Unmanaged debugging | Y | Y | Y | Y |
Managed debugging | | Y | Y | Y |
Remote debugging | Y | Y | Y | Y |
Attach to process | Y | Y | Y | Y |
Detach from process in Win2K and XP | Y | Y | Y | Y |
SQL debugging | N | N | N | Y |
My approach is to learn two debuggers to max my coverage: windbg and visual studio. Visual Studio's design is so good that you don't have to know anything about debugging to use it. So I want to talk a little bit regarding windbg, more specifically, troubleshooting its symbol problems.
PDBs are symbol files. The private variety contains type, local, global, and source line information. So provided that you have the private variety, how do you use them with a debugger to find out which line of the code you are currently executing? First, make sure you read the excellent article written by Saikat Sen at https://www.codeproject.com/KB/debug/windbg_part1.aspx. Instead of describing every step in detail, I will go over how to troubleshoot possible errors encountered regarding symbols.
First thing to try when symbols could not be loaded is to run the .reload command.
If that does not work, you could try to force all modules to reload by doing .reload -f. But chance of this working is low except in only certain situations. Instead we want to enable verbose symbol logging by running !sym noisy. What this does is making the symbol loading portion of windbg spit out more information than normal.
Now, do .reload again. Now you should see all the places that windbg is check for symbols in and how each attempt went. If you actually have the symbols, there are two possibilities that could happen.
Possibility #1: symbols and executable/image does not match. To solve this, either get good symbols or do .symopt+0x40 which forces the usage of the found symbol. This could give you bad info so keep that in mind.
Possibility #2: windbg doesn't look at the folder location that actually has your symbol. To fix this, do .sympath to see the symbol paths it's looking at, then do .sympath+ <path to symbol> to add the symbol directory to what's already there. You can also replace the symbol path by doing .sympath <path to symbol>.
Anyway, that's it for now. Hopefully I'll post more on this area in the future.
Powershell Tip #1
In Powershell, type $profile.
PS C:\Program Files\Microsoft\AxFuzzer> $profile
C:\Users\mengli\Documents\WindowsPowerShell\Microsoft.PowerShell_profile.ps1
That points to where your profile is stored at. This is a powershell script that executes upon the start up of any powershell prompt for the current user. Go ahead and make the file. In my case, I made a new file at the location by typing this:
new-item $profile -itemtype file -force
Now, open the file and you can put in things like this:
set-executionpolicy unrestricted
. \\meng\shared\powershell\hyperv.ps1
set-executionpolicy remotesigned
Every new powershell prompt that you excute will now have executed the hyperv.ps1 file. I've placed mine on a network so I can always load the latest copy on all my computers.
Quick Powershell Tutorial
Not a lot of help text but once you try these commands, you'd learn 90% of the commands most people need from powershell.
Powershell Lab
WMI Object
gwmi win32_service
gwmi -list
gwmi -list | sort-object
gwmi -list -computer switools-vm | sort-object
Quick Detour
gwmi -query "select * from win32_service where name like 'a%'"
gwmi win32_service | where-object {$_.name -like "a*"}
get-process | where-object {$_.name -like "b*"}
Get-EventLog System | Group-Object eventid | Sort-Object Count -descending
Get-EventLog System | Group-Object eventid | Sort-Object Count -descending<enter> | ConvertTo-html | out-file "c:\test\test.html"
Back to WMI
gwmi -list -namespace "root\virtualization" -computer switools-vm | sort-object
(gwmi -computer switools-vm -NameSpace "root\virtualization" -Class "MsVM_VirtualSystemManagementServiceSettingData") | get-member
(gwmi -computer switools-vm -NameSpace "root\virtualization" -Class "MsVM_VirtualSystemManagementServiceSettingData").DefaultVirtualHardDiskPath
Powershell Versus C#
Powershell
$ImgMgtSvc=Get-WmiObject -computerName $server -NameSpace "root\virtualization" -Class "MsVM_ImageManagementService"
$arguments = @($vhdPath,$parentDisk,$null)
$result= $ImgMgtSvc.PSbase.InvokeMethod("CreateDifferencingVirtualHardDisk",$arguments)
C#
ConnectionOptions oConn = new ConnectionOptions();
oConn.Username = "JohnDoe";
oConn.Password = "JohnsPass";
ManagementScope scope = new ManagementScope(@"\\" + server + @"\root\virtualization", oConn);
ManagementObject imageService = Utility.GetServiceObject(scope, "Msvm_ImageManagementService");
ManagementBaseObject inParams = imageService.GetMethodParameters("CreateDifferencingVirtualHardDisk");
inParams["ParentPath"] = parentPath;
inParams["Path"] = path;
ManagementBaseObject outParams = imageService.InvokeMethod("CreateDifferencingVirtualHardDisk", inParams, null);
inParams.Dispose();
outParams.Dispose();
imageService.Dispose();
Functions and Filters
Function Add($x, $y)
{
$x + $y
}
add 5 4
Function FnFilter($name)
{
$input | where-object {$_.name -like "*$name*"}
}
get-process | FnFilter a
for fun: Get-ChildItem -Path C:\ -Recurse | FnFilter temp
Profile
$profile
notepad $profile
Credentials
$cred = get-credential
$cred
$cred|get-member
$cred.password
$cred.password |convertfrom-securestring | set-content c:\temp.txt
$password = Get-Content c:\temp.txt | ConvertTo-SecureString
$credential = new-object system.management.automation.pscredential("redmond\mengli", $password)
.NET
[system.environment] | get-member -static
[system.environment]::GetCommandLineArgs()
[system.environment]::CurrentDirectory
[appdomain]::currentdomain.getassemblies()
notepad++ mymathlib.cs
C:\Windows\Microsoft.NET\Framework\v3.5\csc /target:library mymathlib.cs
mymathlib.cs
namespace MyMathLib
{
public class Methods
{
public Methods()
{
}
public static int Sum(int a, int b)
{
return a + b;
}
public int Product(int a, int b)
{
return a * b;
}
}
}
[Reflection.Assembly]::LoadFile("c:\test\MyMathLib.dll")
[appdomain]::currentdomain.getassemblies()
[MyMathLib.Methods]::Sum(10, 2)
(new-object MyMathLib.Methods).product(10,2)
[System.Reflection.Assembly]::LoadWithPartialName("System.Windows.Forms")
[system.windows.forms.messagebox]::show("bleh")
COM
$comobj = new-object -comobject axfuzz.simple
$comobj.simple(1)
Further Applications
TFS Library from the TFS Power Tools, ActiveDirectory
Recruiting Trip Down in San Diego
Every quarter or so, Microsoft puts together a recruiting trip down to San Diego. The trip usually invovles an hr person with very little technical background accompanied by three or so technical people(PM, SDE, SDET) from various orgs at Microsoft.
The hr recruiter for UCSD contacted me recently and asked if I'd like to accompany him down to UC San Diego for his next trip Feb 19-20th. Although I normally would hate to leave the wonderful winter weather in Seattle, I decided to make an exception in this case. What this particular trip and probably almost all recruiting trip involves is a Microsoft company presentation at night one day and a career fair booth during the day the following day. Over the next few blog posts, I hope to post some tips regarding interviewing at Microsoft or even getting an interview at Microsoft. This will hopefully make my blog more popular, more popular being someone other than me reading it.
Recruiting Trip Down in San Diego part 2
A method that I am using in order to locate top candidate for interviews at Microsoft is by contacting an ex professor of mine, Dr. Ramamohan Paturi. He taught CSE 202, the graduate level algorithm class. In terms of interviewing at Microsoft, it is probably the most useful class taught at UC San Diego. Data Structure, Operating System, and System Architecture come in close behind. Dr. Paturi seems willing to provide me a list of students who might be interested in interviewing at Microsoft. I cannot guarantee them a job, but I can guarantee them an interview, which is fairly precious in this economy.
What this means is that doing well in your classes definitely impacts your career, especially for those who are taking Dr. Paturi's class this quarter. He already assured me that he has some people in mind.
Remotely Managing Hyperv in Win 7
Although, Win 7 official release is this week, internally it has been available for a while now. Remote Management pack apparently came out in August. So if you haven't installed it and you have any hyper v servers, you should get it now! https://www.microsoft.com/downloads/details.aspx?FamilyID=7d2f6ad7-656b-4313-a005-4e344e43997d&displaylang=en#filelist
Tone Matrix the Tenori-on Wannabe
I've been into music a lot lately. And I found a little flash program that is just awesome. It operates very much like the Tenori-on: https://lab.andre-michelle.com/tonematrix. As far as I can tell, the operating principle is such that each square is basically a different LTI system(with very small alterations to the frequency response) and lightning one up allows the program to feed it an impulse function. Then all the impulse response enabled in a particular column is summed up and transformed into a time-domain based signal and added to the currently playing signal. Anyway, ultra cool program. Check it out.
Twitter
I finally signed up for a twitter account: https://twitter.com/softienerd. My first post is on the fact that a social site like groupon is worth more than twitter: https://www.theatlantic.com/technology/archive/2010/09/what-are-your-favorite-social-media-sites-worth/63481/
Visual Studio Load Test Performance Testing
Recently, my team needed to do some perf testing on some ASP.NET application with sql backend. After some research, I discovered two ways to approach the problem that seemed easier than the rest:
Option 1:
Create what's known as web tests within Visual Studio. Plug these into the load test framework in Visual Studio and off you go. Here is a link that will give you more information that I can ever tell you:
https://blogs.msdn.com/b/edglas/archive/2007/12/17/content-index-for-web-tests-and-load-tests.aspx
Pros: Quick and fast. No programming knowledge required at all since everything is down via an action recorder. Basic XML knowledge can help.
Con: Hard to modify.
Option 2:
This is the option that I picked. Write a bunch of unit tests inside Visual Studio using WatiN(https://watin.sourceforge.net/). Then plug the unit tests into the load test framework.
Pros: Quick still, but not as fast as web tests. Customizable just like any other desktop application. Ability to have static initializers that run per performance test run and per performance unit function call. Absolutely necessary if you want to query a database to form an url.
Cons: Not as easy as web test.
Announcing the Release Candidate of Service Pack 2 (SP2) for Windows Vista and Windows Server 2008
As you probably know, the Windows Client team has been developing Service Pack 2 for Windows Vista and Windows Server 2008, representing our commitment to continuous improvement. Last week, on February 25, the Release Candidate (RC) was made available to MSDN and TechNet subscribers; this week we're pleased to announce broad availability under the Customer Preview Program as of today, March 4. Your automated feedback will help us deliver the highest quality final release possible.
SP2 for Windows Vista and Windows Server 2008 builds on the advancements delivered in SP1, and simplifies administration by enabling the deployment and support of a single service pack for client and servers. SP2 includes all of the updates that have been delivered since the release of SP1, along with support for new types of hardware and emerging standards that will grow in importance in the coming months.
A few of the highlights are:
Hardware and Standards support
- Bluetooth 2.1 feature pack to support the most recent Bluetooth device specifications
- Windows Connect Now (WCN) WiFi configuration
- Enhanced exFAT file system to support correct file synchronization across time zones
Performance, Reliability and Security improvements
- All previously released updates since SP1
- Improved wireless reconnection performance for mobile PCs resuming from hibernation
- Reduced audio and video streaming performance for specific hardware configurations
- Windows Search 4, with improved indexing performance, extended file types, and improved Group Policy integration
Deployment and Support enhancements
- A single installer covers both client PC and servers
- Driver detection that will either block SP2 installation or warn users of functionality loss, whichever is more appropriate
- Improved error handling and more descriptive error messages
- Event logging support for administrators who use quiet-mode installations
- Includes the Service Pack Clean-Up tool to restore hard disk space by removing redundant files from RTM and SP1
Many of you also manage server infrastructure. If you are testing out Windows Server 2008 R2, here are a few other things you'll see in this Release Candidate:
- Easier virtualization—Hyper-V RTM is included in the Service Pack
- Reduced power consumption—Windows Server 2008 R2 is up to 10% more power efficient than Windows Server 2008 RTM
- Unified—there is one package for 32 bit and one package for 64 bit that covers both Windows Vista and Windows Server 2008
For more detail on these and other changes in SP2, please check the Windows Team Blog.
Are Your PCs Ready For Windows 7?
When planning for migration to Windows 7, specific knowledge about each computer on the network and its readiness for migration is essential. Manually conducting a detailed network inventory is time consuming and costly; and existing assessment management tools typically require software agent installation on each machine, creating a security risk. How do you gather comprehensive knowledge about your existing IT environment quickly and cost effectively? The Microsoft Assessment and Planning (MAP) Toolkit 4.0 Beta gathers essential infrastructure information to answer the question "are your PCs ready for Windows 7?" This tool also assists you with Windows Server 2008 R2 readiness assessment.
What is the MAP Toolkit?
The Microsoft Assessment and Planning (MAP) Toolkit is a powerful inventory, assessment and reporting tool that securely assesses IT environments for various platform migrations and virtualization without the use of any software agents. This versatile toolkit:
· Quickly discovers clients, servers, and applications across your IT environment
· Conducts migration and virtualization assessment for your IT projects
· Auto-generates reports and proposals
· Scales well to small businesses and large enterprises
Accelerate Your Migration to Windows 7
Quickly and effectively collect the detailed infrastructure information you need for planning your migration to Windows 7. Create a client inventory report, and let MAP 4.0 analyze the data to assess Windows 7 readiness for your unique IT environment.
With MAP 4.0, you get the following reports and proposals for migration to Windows 7:
· Hardware Inventory Proposal. Identify currently installed Windows Client operating systems, detailed analysis of hardware and device compatibility, and recommendations for migration to Windows 7.
· PC Security Report. Identify desktops with anti-virus and anti-malware programs installed and Windows Firewall status.
Register to join the MAP 4.0 Beta program today! (Live ID and registration required.)
REMINDER: Windows 7 Beta expires Aug 1st. Reboots every two hours start July 1st. Upgrade to the Windows 7 Release Candidate today! Click here to download the RC
Beta 1 for Microsoft Enterprise Desktop Virtualization 1.0 (MED-V) is now available
It's been a busy week for beta software downloads—we had a tremendous response to the Windows 7 Beta, to the point where we needed to add server capacity just to keep up! And if you haven't downloaded the Windows 7 Beta yet, you can still do so here.
Right on the heels of Windows 7 is the Beta 1 for Microsoft Enterprise Desktop Virtualization 1.0 (MED-V), one of six technologies in the Microsoft Desktop Optimization Pack (MDOP). MED-V helps to overcome application-to-OS compatibility issues (for example, allowing an application built for Windows XP to operate on a Windows Vista PC), and provides deployment, management and user experience capability for Virtual PC images in an enterprise environment. MED-V is a client-hosted virtualization technology, making it ideal for circumstances where the end user is regularly offline (such as mobile laptop users) or on a limited network connection. The beta is available to the public for free. The final release of MED-V v1.0 will be available through the Microsoft Desktop Optimization Pack—your organization must have or purchase Windows Client Software Assurance on your Windows desktops to buy MDOP.
You can download the MDOP MED-V Version 1 Beta here. Please note—you will need to answer a short five question survey before downloading the software.
For more information on the MED-V Version 1 Beta, there is also an FAQ document and Quick Start Guide available on the download site. And for topical resources on managing a Windows environment, please see the Springboard Series on TechNet.
First Look: Windows Vista Service Pack 2
You might have heard about the upcoming beta release of Windows Vista Service Pack 2 (SP2), and we wanted to take a few minutes to describe what's in it and how it can help you align your plans around Windows Vista adoption. The Windows Vista SP2 beta will be available to a small group of Technology Adoption Program customers on Wednesday, October 29, and we anticipate broad availability for Windows Vista SP2 in the first half of 2009.
It's important to note that this release will embody a single service pack covering both client (Windows Vista) and server (Windows Server 2008), continuing the single serviceability model established with the Windows Vista SP1 and Windows Server 2008 RTM release. This approach helps reduce the testing and deployment complexity for our customers.
In addition to all previously released updates since the launch of Windows Vista SP1, Windows Vista SP2 adds a few new capabilities:
- Support for new types of Hardware and emerging standards, including Bluetooth 2.1, the ability to natively record data on Blu-Ray media, support for ICCD/CCID smart cards, and support for the new VIA 64-bit CPU
- Wi-Fi setup and maintenance is simplified with the addition of Windows Connect Now (WCN)
- The ex-FAT file system is enabled, supporting UTC timestamps to ensure correct file synchronization across time zones
- Windows Search 4.0 is included, which provides users faster and more relevant results. Windows Search 4.0 also allows Group Policy integration, which provides administrative control over search parameters
- End users should also experience improvements in resume performance when a Wi-Fi connection is no longer available
Support for new types of Hardware and emerging standards, including Bluetooth 2.1, the ability to natively record data on Blu-Ray media, support for ICCD/CCID smart cards, and support for the new VIA 64-bit CPU Wi-fi set-up and maintenance is simplified with the addition of Windows Connect Now (WCN) The ex-FAT file system is enabled, supporting UTC timestamps to ensure correct file synchronization across time zones Windows Search 4.0 is included, which provides users faster and more relevant results. Windows Search 4.0 also allows Group Policy integration, which provides administrative control over search parameters End users should also experience improvements in resume performance when a Wi-Fi connection is no longer available
We expect that Windows Vista SP2 will retain compatibility with applications that run on Windows Vista and Windows Vista SP1 and are written using public APIs. Consequently, you can (and should) continue your plans for adopting Windows Vista SP1, and roll SP2 into your deployment image when it becomes available.
One final, but very important note—Windows Vista SP1 is a prerequisite for installing SP2, so you will need to start with a Windows Vista SP1 image before upgrading to SP2.
For more information on Windows Vista for IT professionals, please visit the Springboard Series on the Windows Client TechCenter at www.microsoft.com/springboard.
Got questions for our Windows 7 Springboard Series Virtual Roundtable? Here's your chance.
I wanted to take a moment to offer our blog readers a direct invitation to submit questions for our upcoming Virtual Roundtable Windows 7: To the Beta and Beyond, airing live February 12, 2009 at 11:00am Pacific Standard time. The panel will be answering questions live during the show; any questions we are unable to answer during the show will be posted in a Q&A on our blog following the broadcast.
The Virtual Roundtable panel includes:
Mark Russinovich -- Microsoft Technical Fellow
Mark Minasi -- Technical Writer/Speaker
Erik Lustig -- Microsoft Sr. Product Manager
Jason Leznik -- Windows Product Management Group
Jeremy Chapman -- Windows Product Management Group
Rhonda Layfield -- Enterprise Deployment Expert
Sandeep Singhal -- Microsoft Director/Program Manager
Please submit your questions to vrtable@microsoft.com
Want to know what our virtual roundtables are all about? Check out our September roundtable on Windows Vista performance.
Live from PDC 2008: A First Look at Windows 7
We're here at the Professional Developers Conference (PDC) in Los Angeles, where Microsoft has taken the wraps off the latest client operating system, Windows® 7. The first thing to note is that this is a pre-beta release, and is still an early first-look. While most information out there will focus on how Windows 7 makes everyday tasks easier with improved user experience and productivity scenarios for end users, we thought we'd focus on some first-look information specifically of interest to IT professionals. In this blog, we'll be regularly posting on the challenges and opportunities of upgrading to a modern desktop or laptop client OS with Windows Vista® today, and Windows 7 as more information becomes available through the development lifecycle.
To begin with, the core architecture of Windows 7 remains the same, as it is built on the same foundation as Windows Vista and Windows Server 2008. Our goal is that the majority of applications and devices that work with Windows Vista will work with Windows 7. This is important if you are evaluating or deploying Windows Vista today; in fact, investments in adopting Windows Vista (testing piloting, deploying) will pay off in a smoother transition to Windows 7 when it becomes available.
In designing Windows 7, the engineering team had a clear focus on what we call 'the fundamentals'—performance, application compatibility, device compatibility, reliability, security and battery life. This effort was aided by telemetry data on how PCs are being used and issues that resulted in poor performance or disruption. The focus on fundamentals didn't start with Windows 7; in fact it is the continuation of the work on Windows Vista that materialized in SP1.
So on to the Windows 7 highlights for IT professionals:
Manageability
With Windows Vista we added significantly more parameters that are manageable with Group Policy, such as configuration and control over Power settings; with Windows 7 we are now extending the reach of what Group Policy can manage, and how settings are applied to specific users or computers, including non-GP aware components.
Updating mobile PCs that spend most of their time off the network is a particularly challenging issue for IT organizations. Windows 7 will introduce DirectAccess, a capability that allows IT to manage and update internet-connected remote PCs, even when they are off the corporate network, while giving mobile users seamless secure connectivity to the corporate resources from the road without having to initiate VPN connection. Also for IT pros, the new Powershell v2 and its graphical editor help automate repetitive tasks with minimal scripting expertise required.
Security and Compliance
Windows Vista improved manageability and security, in particular, by limiting changes to the system without administrative credentials and making it easier to adopt the standard user mode. The introduction of BitLocker® Drive Encryption in Windows Vista, and the extension of this protection to non-boot volumes in SP1 provided the protection of confidential data required in many industries.
Windows 7 builds on these advancements with customizable User Account Control (UAC) that allows IT pros to "tune" the feature based on their environment. For data protection, Windows 7 introduces BitLocker To Go, extending encryption to removable drives. This feature gives greater control over information leaving the corporation, as well as protecting lost or stolen USB drives. Windows 7 also allows IT to control access to specific applications by specific users, but we'll cover these in more detail in future blog posts.
Deployment
Windows Vista delivered significant deployment improvements based on Windows Imaging Format (WIM) that allows a hardware and language-independent image to be created and deployed. In many instances, a single image could be deployed and maintained worldwide, providing a more predictable environment. Several new tools, including the Microsoft® Deployment Toolkit, the Application Compatibility Toolkit, and Microsoft Assessment and Planning toolkit helped streamline the planning, testing and deployment of a large-scale deployment.
In Windows 7, image creation and deployment is enhanced with advances such as Dynamic Driver provisioning, the Deployment Image Service and Management tool, Multicast Multiple Stream Transfer, and improvements to user state migration. We'll go into further detail in future blog posts, so check back frequently.
In conclusion, Windows 7 promises advancements in manageability, security, deployment and end user productivity. Does this mean you should wait or skip Windows Vista? The fact is that you can get the many of the advantages today in Windows Vista. Much progress has been made with Windows Vista SP1 and a maturing ecosystem, and this progress continues with Windows 7.
If your organization hasn't begun looking seriously at Windows Vista, or if you evaluated Windows Vista prior to SP1, it now makes sense to re-evaluate—both to benefit from more advanced PC environment, and to get ahead of the adoption curve for Windows 7.
To learn more about Windows 7, Windows Vista or any of the Windows Client technologies, please visit www.microsoft.com/springboard for the latest in information, guidance and community connections.
Popular posts from this blog
视频教程和截图:Windows8.1 Update 1 [原文发表地址] : Video Tutorial and Screenshots: Windows 8.1 Update 1 [原文发表时间] : 4/3/2014 我有一个私人的MSDN账户,所以我第一时间下载安装了Windows8.1 Update,在未来的几周内他将会慢慢的被公诸于世。 这会是最终的版本吗?它只是一项显著的改进而已。我在用X1碳触摸屏的笔记本电脑,虽然他有一个触摸屏,但我经常用的却是鼠标和键盘。在Store应用程序(全屏)和桌面程序之间来回切换让我感到很惬意,但总是会有一点瑕疵。你正在跨越两个世界。我想要生活在统一的世界,而这个Windows的更新以统一的度量方式将他们二者合并到一起,这就意味着当我使用我的电脑的时候会非常流畅。 我刚刚公开了一个全新的5分钟长YouTube视频,它可以带你参观一下一些新功能。 https://www.youtube.com/watch?feature=player_embedded&v=BcW8wu0Qnew#t=0 在你升级完成之后,你会立刻注意到Windows Store-一个全屏的应用程序,请注意它是固定在你的桌面的任务栏上。现在你也可以把任何的应用程序固定到你的任务栏上。 甚至更好,你可以右键关闭它们,就像以前一样: 像Xbox Music这种使用媒体控件的Windows Store应用程序也能获得类似于任务栏按钮内嵌媒体控件的任务栏功能增强。在这里,当我在桌面的时候,我可以控制Windows Store里面的音乐。当你按音量键的时候,通用音乐的控件也会弹出来。 现在开始界面上会有一个电源按钮和搜索键 如果你用鼠标右键单击一个固定的磁片形图标(或按Shift+F10),你将会看到熟悉的菜单,通过菜单你可以改变大小,固定到任务栏等等。 还添加了一些不错的功能和微妙变化,这对经常出差的我来说非常棒。我现在可以管理我已知的Wi-Fi网络了,这在Win7里面是被去掉了或是隐藏了,以至于我曾经写了一个实用的 管理无线网络程序 。好了,现在它又可用了。 你可以将鼠标移至Windows Store应用程序的顶部,一个小标题栏会出现。单击标题栏的左边,然后你就可以...
ASP.NET AJAX RC 1 is here! Download now
Moving on with WebParticles 1 Deploying to the _app_bin folder This post adds to Tony Rabun's post "WebParticles: Developing and Using Web User Controls WebParts in Microsoft Office SharePoint Server 2007" . In the original post, the web part DLLs are deployed in the GAC. During the development period, this could become a bit of a pain as you will be doing numerous compile, deploy then test cycles. Putting the DLLs in the _app_bin folder of the SharePoint web application makes things a bit easier. Make sure the web part class that load the user control has the GUID attribute and the constructor sets the export mode to all. Figure 1 - The web part class 2. Add the AllowPartiallyTrustedCallers Attribute to the AssemblyInfo.cs file of the web part project and all other DLL projects it is referencing. Figure 2 - Marking the assembly with AllowPartiallyTrustedCallers attribute 3. Copy all the DLLs from the bin folder of the web part...
Announcing the AdventureWorks OData Feed sample
Update – Removing Built-in Applications from Windows 8 In October last year I published a script that is designed to remove the built-in Windows 8 applications when creating a Windows 8 image. After a reading some of the comments in that blog post I decided to create a new version of the script that is simpler to use. The new script removes the need to know the full name for the app and the different names for each architecture. I am sure you will agree that this name - Microsoft.Bing – is much easier to manage than this - Microsoft.Bing_1.2.0.137_x86__8wekyb3d8bbwe. The script below takes a simple list of Apps and then removes the provisioned package and the package that is installed for the Administrator. To adjust the script for your requirements simply update the $AppList comma separated list to include the Apps you want to remove. $AppsList = "Microsoft.Bing" , "Microsoft.BingFinance" , "Microsoft.BingMaps" , "Microsoft.Bing...
Comments
Post a Comment